Vue table with select row:A Vue table with select row allows users to select one or more rows from a table. This can be useful for performing actions on selected rows, such as deleting or editing them. The implementation typically involves adding a checkbox or radio button to each row, and keeping track of the selected rows using a data property or Vuex store. When the user selects a row, the corresponding data is updated, and the table UI reflects the selection state. This can be further enhanced by adding pagination, sorting, and filtering functionality to the table, making it a powerful tool for displaying and manipulating large sets of data in a web application.
How can I implement a Vue table that allows selecting rows and highlighting them for further actions?
This is a Vue table that allows selecting rows and highlighting them for further actions.
The table is created using a v-for
loop that loops through the items
array and displays each item’s id
, name
, and email
in a table row.
The :class
binding is used to add the selected
class to a row when it is selected. The isSelected
method checks if a row is in the selectedRows
array and returns a boolean value that determines whether the row should be highlighted or not.
The toggleSelected
method is called when a row is clicked. It checks if the row is already selected, and if not, it adds it to the selectedRows
array. If the row is already selected, it removes it from the selectedRows
array.
The selectedRows
array keeps track of the selected rows, and you can use it to perform further actions on those rows.\
Vue table with select row Example
<div id="app">
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr v-for="item in items" :key="item.id" :class="{ 'selected': isSelected(item) }"
@click="toggleSelected(item)">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.email }}</td>
</tr>
</tbody>
</table>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
items: [
{ id: 1, name: 'John', email: 'john@example.com' },
{ id: 2, name: 'Jane', email: 'jane@example.com' },
{ id: 3, name: 'Bob', email: 'bob@example.com' },
{ id: 4, name: 'Andrew', email: 'andrew@example.com' },
],
selectedRows: [],
}
},
methods: {
isSelected(item) {
return this.selectedRows.indexOf(item) !== -1;
},
toggleSelected(item) {
const index = this.selectedRows.indexOf(item);
if (index === -1) {
this.selectedRows.push(item);
} else {
this.selectedRows.splice(index, 1);
}
},
},
});
app.mount('#app');
</script>
Output of Vue table with select row
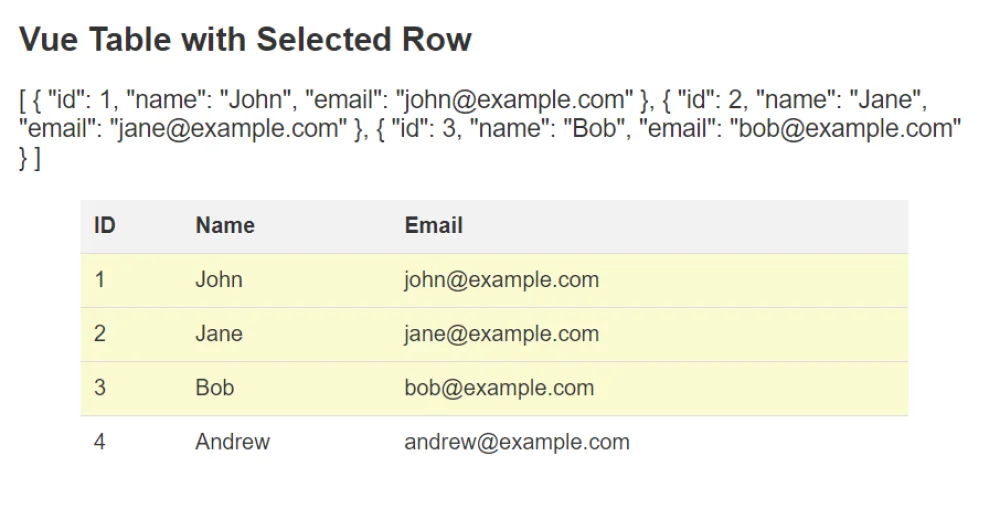